Home > backend > How to Build a Powerful RESTful API with Go (Gin-web) and Supabase.
How to Build a Powerful RESTful API with Go (Gin-web) and Supabase.
Build a powerful and scalable RESTful API with Gin-Golang and Supabase.
By :Thomas Inyangđ 24 Jan 2025
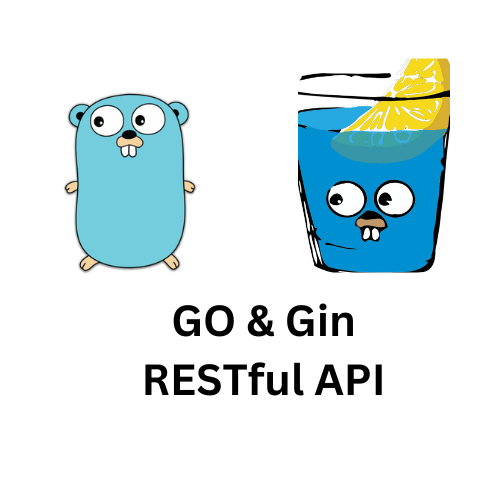
Introduction
If you are a backend developer who wants to build a fast and scalable RESTful API that performs Create Read Update and Delete (CRUD) operation, then this post is for you.
In this post, you'll learn the following:
- How to set up Gin in your Golang environment.
- Make requests using the common HTTP request methods.
- Set up a supabase account and connect it with the project.
- Build a RESTful API.
Prerequisites
- Install GO.
- A supabase account.
- Internet connection.
REST is an acronym for Representational State Transfer, while API is an acronym for Application Programming Interface.
RESTful API is an interface (medium) that connects two or more computers to exchange information locally or remotely over a secure network and it has the following features:
- Common HTTP request methods (GET, PUT, POST, DELETE).
- Platform independence for various programming languages and devices and it also supports multiple data formats (JSON, XML, plain text).
- It is scalable through stateless horizontal scaling and improves performance with cachingâby storing responses for reuse, reducing server load and latency.
- It also offers security with OAuth, and SSL/TLS encryption and allows versioning to maintain backward compatibility without disrupting existing clients.
Gin is a Go (Golang) web framework that is tested for its speed, scalability, and ability to efficiently handle concurrent requests. When you want to build a lightweight but powerful API that is scalable, Gin-Golang should top your options list.
Let's get started.
How to Create Your First RESTful API with Gin-Golang.
In this section, you will learn how to set up the Gin-Golang environment to build a scalable RESTful API.
Step1
Create a folder (go-backend) in the desktop directory and open it with your code editor (VSCode).
Step2
In the code editor, open the terminal ctrl + ` and enter the following code.
This code will create a main.go file (the API entry point), initialize the API project, and install Gin package.
Step3
Enter this code in the created main.go file.
In this code, gin is used to set up the HTTP Server which returns a âHello World!â message when the route "/ping" is tested on HTTP GET request header.
In the terminal, enter go run . to start the local server.
Step4
In your code editor, install Thunder Client extension, this will enable you to test the endpoints.
Launch the Thunder Client extension, select GET as the head type, enter the url http://localhost:10000/ping, and hit SEND. This will return a response
{
"message": "Hello World!"
}
Step5
In the root directory of the project, create a controller folder (local module) with a file controller.go, this is where your file will contain all the REST API functions and logic.
See Also: How to Create Local Modules in Golang.
Open the controller.go file in the integrated terminal (right click on the file and select 'open in integrated terminal'), when it's opened, install gin.
go get -u github.com/gin-gonic/gin
After gin is installed successfully, enter this code in the controller.go file.
After entering this code, run these commands in the terminal of the controller file.
go mod init example.com/yourSytemUsername/controller
go mod tidy
This initialises the controller file as a local module and ensures all imported package(s) are in the project directory. The Ping handler (local module) is ready for use.
Step6
Usage: to use this Ping handler (local module), open the main.go file in the terminal (right click on it and select âOpen in Integrated Terminalâ option) and enter
When this is done,update the main.go file with this code.
You can see that this makes the entry file (main.go) readable and easy to maintain.
If you experience some compile time error, move your cursor to the line of code and select the quick fix from the popup. When you're done and there is no more compile time error, let's create the handlers for the scheduler RESTful API.
How to Create a RESTful API Handler Function in Gin-Golang.
As required, you should have your supabase anon key and URL, if you've not done that, you need to.
In your supabase dashboard, Create a scheduler table (it should be public) with the following columns, description, day_month, and priority.
Click on the "Add RSL Policy " to create a policy for the scheduler table.
When redirected, click on " create policy".
When a new page opens, enter the following policies.
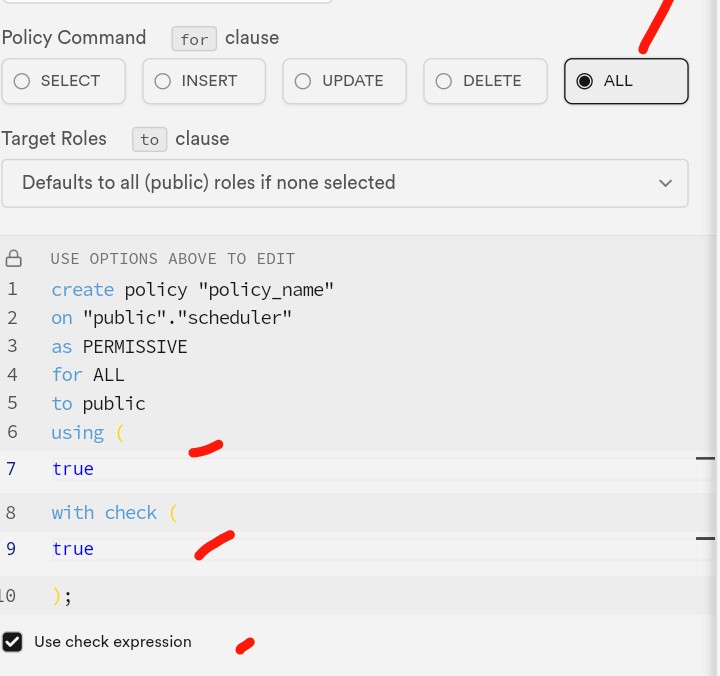
This will enable you Create(Insert), Read(Select), Update, and Delete a schedule from the scheduler Table.
Now that you've created the supabase instance and added a RSL policy on your scheduler Table, you can go ahead to initialise the connection and create the handlers.
Step1
Create a .env file in the project folder (folder/.env) and enter your supabase url and anon key.
SUPABASE_URL = https://***
SUPABASE_ANON_KEY =***
Step2
In the controller.go file, install the following via the controller.go terminal,
go get github.com/joho/godotenv and
go get github.com/supabase-community/supabase-go.
This will install both the .env and supabase package.
Update the controller.go file with this code
In this code,
- godotenv.Load() loads the supabase URL and Anon-key from the .env file.
- The supabase method is initialised , if there is an error in initialising the supabase method, it prints the error, else it returns the client instance
Step3
In the controller.go file, create a struct type to create a schedule.
```
In this code, the database columns are represented in JSON with the binding as "required". When binding is enforced, the field must not be an empty value, else, an error will be returned.
Also, create the CreateSchedule handler.
In this code:
- Youâve defined the CreateSchedule handler.
- The supaClient stores the returned instance from the ConnectDB function.
- You declared a json variable with schedule as its Type. This is used in the ShouldBindJSON which ensures that what is declared in the schedule struct type is enforced. When the value is empty or a wrong data type is entered, an error is returned.
- row := schedule{...} is the placeholder with data for the scheduler DB columns.
- _, _, err := supaClient.From("scheduler").Insert(row, false, "", "", "").Execute(). This is used to insert (create) a schedule into the scheduler DB. The row variable represents the columns of the scheduler DB.
- If there is an error during the insertion process, an "internal server error" error message is returned, if there is none, a success message â "created" is returned.
Step4
Create a structure type to get all schedules.
This GetSchedule struct type is different from that of schedule, in this case "binding: required" is not used since you'll not be entering any data.
Enter the function
This code gets all the schedules from the DB and Unmarshal them into allSchedules variable.
See Also: How to Test RESTful APIs.
Step5
This code will delete a schedule based on the schedule id.
Step6
Handler to Update a Schedule
This will update a schedule based on the id.
Step7 .
You can now use the handlers function (local modules) you created in the controller.go file.
In the main.go file, update the existing code with this.
With this code, your backend server is set.
In the main.go terminal, enter this code go run .
Step8
You can try out the endpoints using the thunder client extension.
To create:POST http://localhost:10000/createSchedule
To update:PATCH http://localhost:10000/updateSchedule?q=11
To delete :DELETE http://localhost:10000/deleteSchedule?q=0
Where q is the query key, 11 and 0 are the id of the schedule to be updated and deleted.
The above steps are not exhaustive, you can include other functions based on your preference.
See Also: How to Set Up Cookies in GO Using Gin-Web
Conclusion.
This post explains how to create a strong REST API with Gin, a popular Golang web framework, and Supabase.
It also covers how to set up Row-Level Security (RLS) policies for your database tables, create reusable Golang modules (local functions), and test your API endpoints with Visual Studio Code's Thunder Client plugin.
Please Share.