Home > Edu-Tech > DDA Algorithm: How To Draw a Smooth Line Using Python PL Packages- Numpy and Matplotlip
DDA Algorithm: How To Draw a Smooth Line Using Python PL Packages- Numpy and Matplotlip
How to use numpy and matplotlip to draw a DDA smooth line.
By :Thomas Inyang🕒 14 Jun 2024
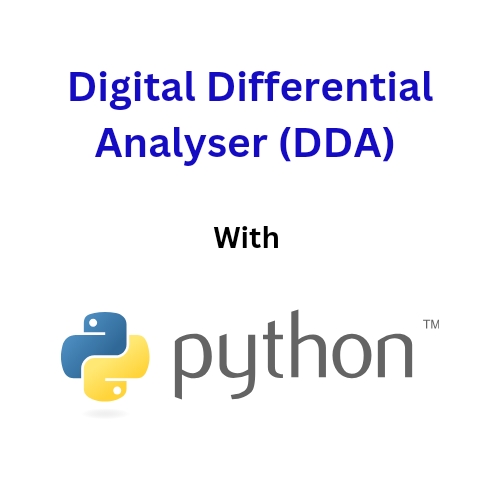
Brief Concept of Computer Graphics.
Graphics are the cornerstone of a smooth and engaging user experience in computing. They go beyond creativity; they play a crucial role in effective communication and understanding. Icons, illustrations, charts, and animations all contribute to the functionality and overall appeal of software applications, websites, and digital media.
Understanding Line Drawing.
Drawing a perfect line on a computer screen might seem simple, but it's an approximation due to the pixel-based nature of displays. Line drawing algorithms come into play here, acting as a bridge between the ideal line concept and its digital representation. These algorithms determine which pixels to color to create the best visual representation of a line segment.
What is a Digital Differential Analyzer (DDA)
The Digital Differential Analyzer (DDA) is a popular line-drawing algorithm used in computer graphics. It's known for its simplicity and efficiency. DDA works by calculating the incremental difference between the starting and ending points of a line segment, both in the x and y directions. This information is then used to determine which pixels to color as the line is drawn.
Break down the DDA algorithm steps:
- Calculating the difference in x and y coordinates (dx, dy) between the starting (x0, y0) and ending points (x1, y1).
- Determining the number of steps needed to draw the line.
- Calculating the increment values for x and y coordinates in each step (Xincrement, Yincrement).
- Iteratively calculating and plotting points along the line based on the increment values.
Implementing DDA Algorithm with Python.
This code block contains the sequential steps for implementing Digital Differential Analyzer (DDA) line.
Conclusion
The DDA algorithm is known for its simplicity and efficiency, calculates the incremental change in coordinates to draw lines in computer graphics. While it has applications in various graphics tasks, its ease of implementation in Python makes it a valuable learning tool. However, for smoother lines, especially at steeper angles, exploring algorithms like Bresenham's line algorithm is recommended.
Please Share