Home > software_development > Golang local module package implementation guide
Golang local module package implementation guide
A detailed example of creating and using Golang local module.
By :Thomas Inyang🕒 30 Jun 2024
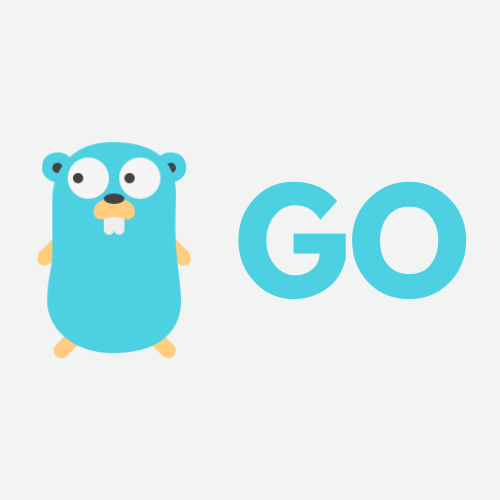
This post is for developers who use Go programming language for web development and would like to create and use reusable functions (local packages). Before you create and use local packages, you need to have a better understanding of the answer provided in the question below.
Why do developers create reusable functions?
Developers create reusable functions to store a piece of code that does a single task inside a defined block and then calls that code whenever they need it using a single short command, rather than having to type out the same code multiple times. Also, code reusability can improve code quality and maintainability by making it easier to identify and fix bugs at a later time.
How to create a local module to be reusable across all Golang folder structures.
In Go, you can create a function as a local module and it can be used as a package where it's needed.
Assuming you have an existing project or you can create a new one from the desktop directory.
Step1
- Create a folder, maths.
- Open the folder with the code editor (right-click on the folder and select "open with code").
- In the maths folder, create a file (main.go) and enter this code.
The above is the entry code structure for Golang and it can only be executed when the main.go file is initialized.
To initialize the main.go file, open the main.go directory in the terminal and run the following
go mod init is used to initialize the main.go file, while go mod tidy is used to tidy the file to run successfully without errors.
When this is done successfully, you can run go run main.go which will then print Hello World.
Step2
Let's create the reusable functions folder, in this case, let's name it formula
- In the formula folder, create a file function.go
- In the file, create the reusable functions to calculate the area of a circle and triangle.
maths/formula/formula.go
The local package name is formula, the func AreaOfCircle will accept an argument radius and return the area, while the func AreaOfTriangle will accept two arguments, base and height, and return the area.
Open the formula.go directory in the terminal and initialize it by running the following,
go mod init is used to initialize the main.go file, while go mod tidy is used to tidy the file to run successfully without errors.
Step3
You can now use the formula module in the main.go file.
Open the main.go file in the terminal and run the following.
go mod edit -replace replaces initialize directory of formula to "./formula" while go mod tidy is used to tidy the file to run successfully without errors.
In the main.go file, import the local module, and start using it.
To see the functions in action, open the main.go file in the terminal and enter "go run ." The dot is part of the command.
Conclusions
In this post, you've had a better understanding of why you need to create reusable functions and how to create and use one in Golang.
The above steps are the basics of creating a local module that can be used in any file of a folder.
If the local module is to be used in a file of a nested folder, add an extra dot
e.g $ go mod edit -replace example.com/your system username/formula=../formula
If you find this article interesting, please share.