Home > software_development > How to Use JavaScript Events with HTML Elements.
How to Use JavaScript Events with HTML Elements.
JavaScript Events are used to make a web page interactive.
By :Thomas Inyang🕒 13 Sept 2024
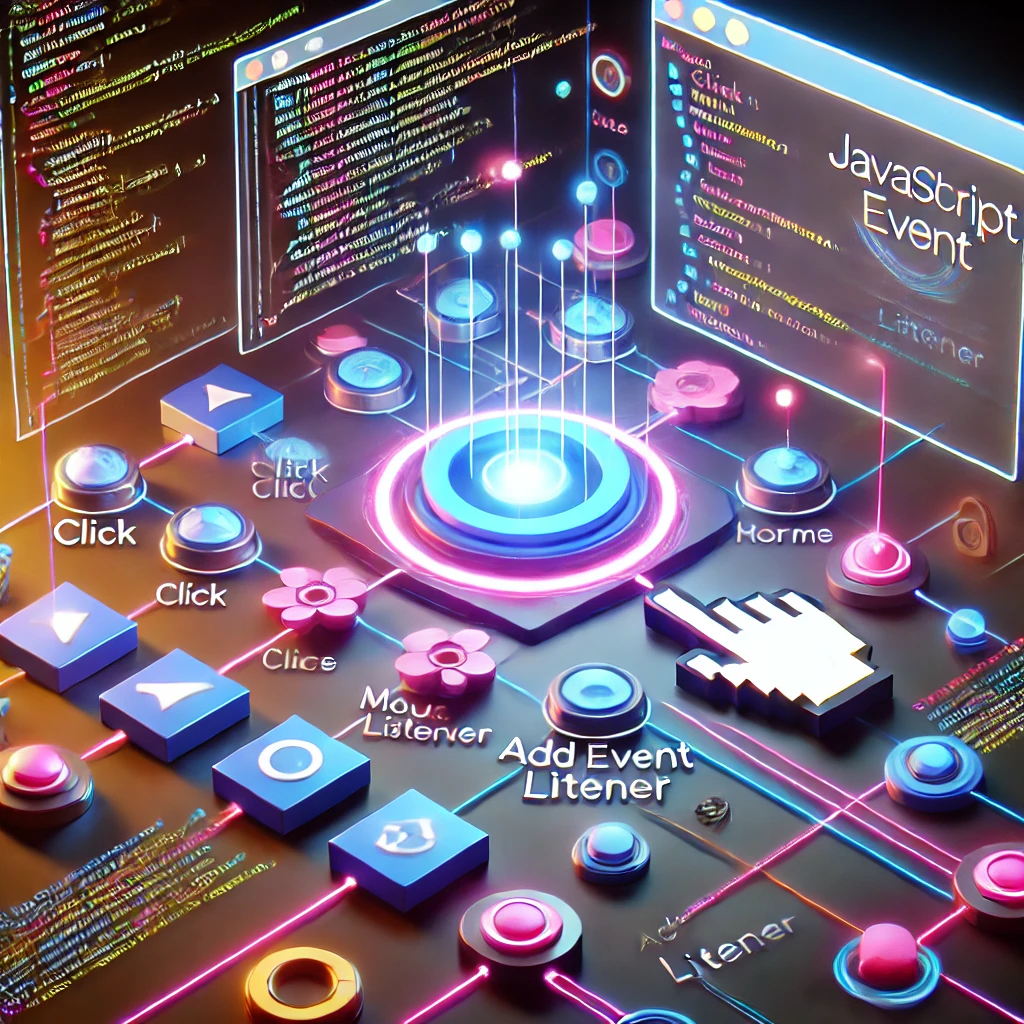
In this post, you will learn about JavaScript events and how they interact with HTML elements, how to write and handle an event in JavaScript.
Introduction.
JavaScript is a dynamic programming language that is widely used for frontend development scripting. It can be used to write and add event functions to any HTML element - body, H1-H6, p, a, button, form, etc.
What are JavaScript events?
Events are things that get executed on a webpage during and after development and JavaScript can react to the events. An event can be placed in one or more elements and it can be loaded in the current tab or affects the entire browser.
The event triggers a function(s) based on the following:
- When a web page loads completely.
- When an element is clicked.
- When a mouse hovers over an element content.
- When an input field value changes.
- When a web page is scrolled up and down.
- Submit a web form, etc.
Other events can be triggered on the web page via the browser.
Here is a basic inline structure of how an event is added to an HTML element, <element event="JavaScript function">.
In the code above, button is the HTML element, onclick is the event, and Date() is the JavaScript function. When the button content - "The time is?" is clicked, the current time and date value is passed to the p element with id "example" for display.
Ways to Handle JavaScript Events.
Events can be handled in 3 different ways
- addEventListener("event type", function).
- on"event type", i.e. onclick, onchange - element.onclick = function.
- Inline - <div onclick="bgChange()">Press me</div>
These methods are valid for handling events in JavaScript.
There are ways you can write your JavaScript event functions inside the <script> element at the end of the body tag, as an external JavaScript file, or as an inline function.
In this post, you'll write the JavaScript event handler at the end of the body tag.
Placing the event handler at the end of the body element allows the page to load completely before the event starts interacting with the HTML elements.
How to add JavaScript addEventListener.
The addEventListener() method is the recommended way to register an event listener, it accepts the following: arguments, event type, function or an object, and options (optional). The addEventListener is then attached to the specified EventTarget (HTML element). If a function or object is already on the list of event listeners for this target, it is not added again.
Let's see how the addEventListener() method works in the example below.
const button is used to target the button element with the id "myButton", which is then used to connect the addEventListener. "click" in the addEventListener is the event type, the function is anonymous "()=>{ }", and alert is a JavaScript predefined function that shows the text 'Button Clicked' when a user clicks the "Click Me" text. This is the basic way to use addEventListener, you can also name your function instead of it being anonymous,
In this method, the function is no longer anonymous, it has a name - showMessage.
Using addEventListener comes with the following benefits.
- It works on any event target, SVG (Scalable Vector Graphics) inclusive.
- You can add more than one handler for an event.
- Provides more control over event propagation and handling.
The use of addEventListener() to register event handlers is the most effective method that scales best with more complex programs. Also, you can remove an event listener with, removeEventListener(event type, function, options). Let's see another way to add an event.
HTML Event Attributes.
A button tag has event attributes that can trigger a Javascript function, these attributes are written as on followed by the name of the event type (onclick). You can assign any handler function to an event.
For example, you can change a button element background color like this:
When the page loads completely and "Change my color" is clicked, the background color changes from white to blue.
The event onclick fires the function changeBgColor which changes the button background color. JavaScript events can change the style of an HTML element that's why we used document.querySelector("button").style.background = "blue" to target the button tag and change the background color to blue, if there are more button tags, target the exact button by using getElementById("specify the id"), style.background is a HTML DOM property.
The drawback of the "onclick" method is that you can not add more than one handler for a single event.
The next handler to consider is the inline event handler.
See Also : How to Fetch Data With Javascript Fetch API.
How to use an Inline Event Handler.
This is an example of an inline event handler.
In this example, the onclick event handler fires the predefined function (alert) when the user clicks on "Press me".
Using this method is considered bad practice because the function is not separated and in the long run it becomes difficult to manage due to its poor readability. The best practice is to have a separate JavaScript section - placed at the end of the body element or have an external Javascript file.
Where to Place an External JavaScript File.
You can place an external script reference in <head> or <body> as you like.
When it's placed in the <body>.
The src attribute is used to locate the external Javascript file path. In some cases, the file path might be in a folder, nested folder, or an absolute file path.
Placing an external Javascript file at the end of the body tag is a good practice and it comes with the following advantages.
- The Javascript event functions are separated for the HTML.
- It is easy to read and maintain the HTML and a Javascript file.
- The load time becomes faster when the Javascript file is cached.
It's advisable to write your Javascript in a separate file if you intend to write large Javascript event functions.
Conclusion.
For your webpage to be interactive you need to register an Event that triggers a Javascript function.
The Event (event handlers) can be registered through the following
- Directly in the HTML element
- Using the "on event type" (onclick) attribute in the HTML element to execute a Javascript function.
- Using addEventListener which targets the HTML element that executes the Javascript function.
The event handlers and functions are written in between the <script> tag and can be placed In the <head> or <body> tag. Placing the script tag at the end of the </body> tag is recommended as it allows the HTML elements to load first before interactions can take place.
If the Javascript event and functions will be large, it's recommended you create a separate Javascript file (demoScript.js), reference the path, and place it at the end of the body tag of the HTML file, " <script src="demoScript.js></script>".
I believe you've been able to learn a thing or two about event handlers in Javascript.
Please share