Home > frontend > NextJs Auth: How to Implement OAuth in Your NextJs App
NextJs Auth: How to Implement OAuth in Your NextJs App
Implementing NextJs Auth with ease. Leveraging Google OAuth of Firebase Authentication.
By :Thomas Inyangđ 1 Feb 2025
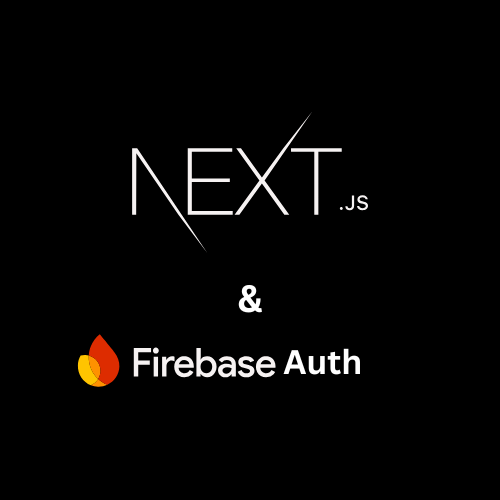
Introduction.
OAuth 2.0 (Open Authorization) is an industry-standard authorization mechanism. OAuth 2.0 promotes client developer simplicity by providing specific authorization processes for web apps, desktop applications, mobile phones, and home entertainment devices that do not require you to create a new account.
Most frontend developers working with Next.js (App Router) frequently find themselves at a crossroads when it comes to choosing an authentication mechanism that is simple to implement, improves user experience, and handles data quickly. As a result, this guide post is written to satisfy those demands and provide a straightforward, step-by-step solution.
In this guide post, you will learn how to do the following:
- Use firebase-auth OAuth to authorize and manage users in your NextJs App.
- Create an AuthProvider that will be used to wrap the root layout.
- Create a Google sign-up/log-in button.
What you need to know for this guide post.
Prerequisite.
- A firebase account.
- A new/existing NextJs project.
- Context provider (useContext)
- React useEffect hook
Letâs begin!!!
How to Setup a Firebase Authentication Account.
In this section, you will learn how to set up a firebase account--authentication for the first time.
Step1.
- Click on Get Started option On the firebase website.
- Select authentication.
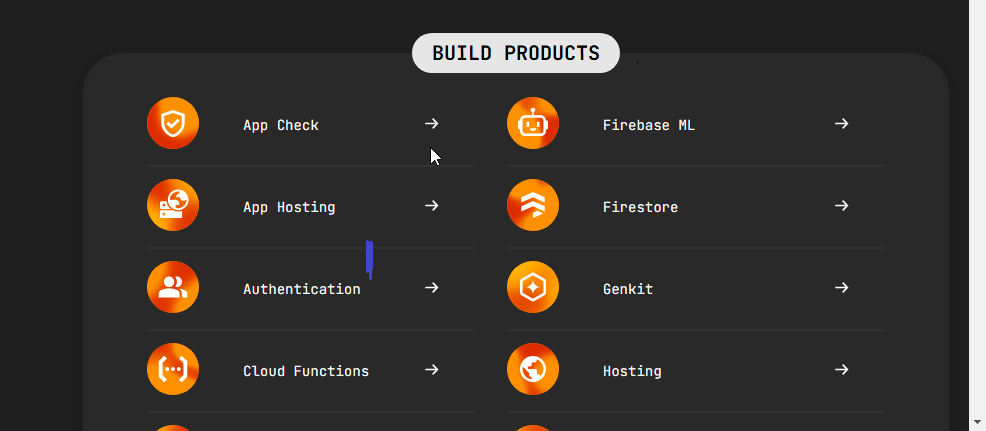
- You will be redirected to a page, click on Create a project.
- On the next page, Enter your project name then click continue.
- On the next page, choose web and you will be provided with your project config value. Copy and save somewhere.
Having done all of these, open your Nextjs app with your favorite editor. In this case, VScode.
Step2.
When your auth dashboard is up do the following:
- At the sidebar, click on the authentication and when redirected.
- Click on âsign-in methodâ, click on add provider too, and select Google.
Google Oauth is now ready for implementation.
How to Implement Firebase OAuth in NextJs Project (App Router).
This section will cover the detailed setup of OAuth in a NextJs project. Also, youâll often use the âuse clientâ flag â a crucial feature in Next.js App Router to specify which components should be rendered on the client side, enabling interactivity and state management that requires browser-side JavaScript.
Step1.
Install firebase
Step2.
In your project dir, create a config folder with a firebaseConfig.js in it and enter your Firebase App object.
See Also: How to Fetch Data Using Javascript Fetch Method.
Step3
In the pages dir, create a signUp folder and a page.tsx file.
In this code,
- youâve imported the necessary instances from firebase/auth, the configuration that contains your firebase auth object, and useRouter from next/navigation.
- Initialized router from useRouter()
- The auth language is set to english.
- A googlePop() function is created containing all the logic that is needed to run the Google OAuth effectively. When there is a user the user is redirected to a desired page, in this case â/dashboardâ.
- A button is returned and when clicked, the Google popup is invoked.
After this, create a context that gets the users' profile which will be globally available.
Step4.
Create a context folder and authContext.jsx in it.
app/context/authContext.js
In this code, you used createContext and useContext of react to create a global state for signed-up users. This code checks if there is a user, if yes, the userâs displayName, email, and photoURL are extracted for use.
Step6.
Create a file ProtectRoutes.jsx in the components folder and enter this code.
This code checks if the user is logged in when the user tries to access a protected page, if the user is not authorized the user is directed to the login page. Also, this code will be used to wrap the protected paths in the next section.
Step6.
Create a file ProviderWrapper.js in the context folder.
app/context/providerWrapper.js
This code is created because in NextJs using the "use client " flag in the rootLayout is not visible. This code will then be used to wrap the entire project layout making the verification and authorization of users globally available and also enforcing the paths protection.
This is how it works.
- Specified paths that are not protected (openRoutes variable).
- Include children as a destructed prop, since it'll be used to wrap the children's prop in the rootLayout.
- A condition is initialized, and it checks if the active path (pathname) is included in the openRoute if yes, that path is accessible, else the ProtectRoute component is invoked âchecks the logged-in user and grants access or redirects to the login page.
- You'll notice that the AuthContextComp is used to wrap the open and protect paths (route) making the logged-in user details globally available.
See Also: How to Implement Free Analytics in Your NextJS App
Step7.
Update the rootLayout file: app/layout.tsx
In this file, the Provider component from the previous step is used to wrap the children prop.
With this done, unauthorized users cannot access protected paths, and logged-in usersâ details can be accessed anywhere in the app. Letâs see an example.
How to use React useContext in a Nextjs App.
Be reminded that, for you to use any of the React hooks in a Nextjs file, you must include the âuse clientâ flag. Assume you have a file in this dir
app/pages/dashboard/page.jsx/tsx
In this file, you imported the useUser from authContext, also the userâs displayname is now globally accessible because you wrapped the Provider component around the children prop in the app/layout.jsx/tsx file.
Finally, save your files and run.
Conclusion
In this guide, you learn how to use Google OAuth in a Next.js application.
I offered a step-by-step guide for creating a Firebase account and establishing Google as the authentication provider.
We also looked at how to successfully implement Google OAuth into a Next.js project, assuring a pleasant authentication experience.
Have you tried this method before? Share your experience in the comments!
Please share.